Plot cifti from ROIs#
[1]:
import nibabel as nib
import pandas as pd
import numpy as np
from neurotools.plotting import plot
from neurotools.loading import load
from neurotools.transform import SurfLabels
import matplotlib.pyplot as plt
The first step is loading some set of rois for an example subject just as a 1D numpy array, in this case w/ length 379.
[2]:
rois = pd.read_csv('ex_rois.csv', header=None)
rois = np.squeeze(np.array(rois))
rois.shape
[2]:
(379,)
Next, we need to load the parcellation label file in a simmilar way, i.e., as just a flat 1D array.
[3]:
parcel = nib.load('HCP2016FreeSurferSubcortical_dparc.dlabel.nii')
parcel_labels = np.array(parcel.get_fdata()).squeeze()
parcel_labels.shape
[3]:
(96854,)
Then, we initialize a SurfLabels object based off the parcel labels file - which we can use to inverse transform the ROIs back into the space of the dlabel file
[4]:
sl_transformer = SurfLabels(parcel_labels, background_label=0)
data = sl_transformer.inverse_transform(rois)
[5]:
plot(data)
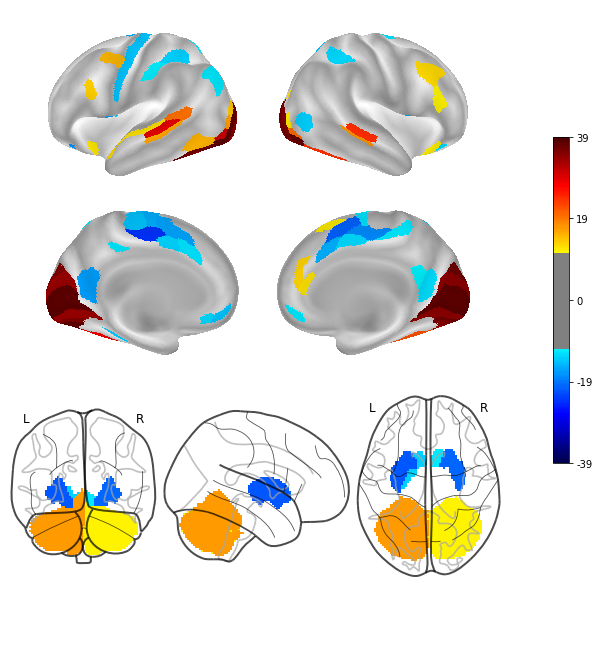
[6]:
plot(data, vol_plot_type='stat', title='My Data')
/home/sage/anaconda3/envs/bpt/lib/python3.9/site-packages/nilearn/plotting/img_plotting.py:348: FutureWarning: Default resolution of the MNI template will change from 2mm to 1mm in version 0.10.0
anat_img = load_mni152_template()
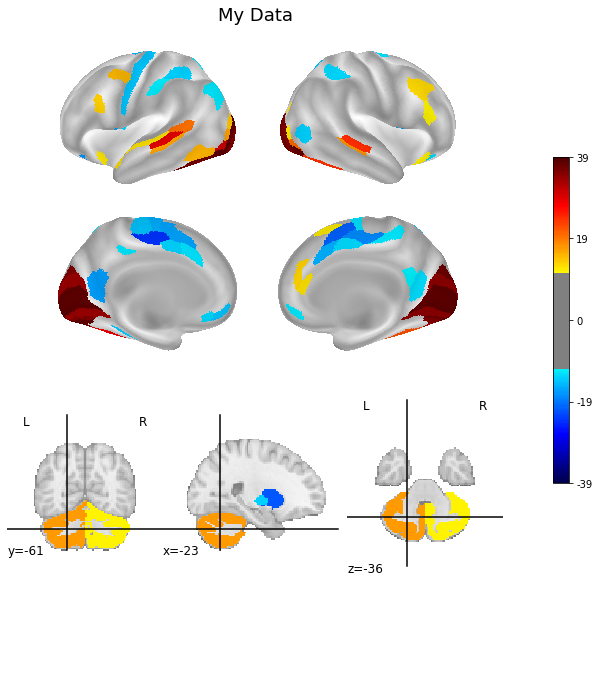
[7]:
# You can also plot the parcellation
# with the same command! Wowza
plot(parcel)
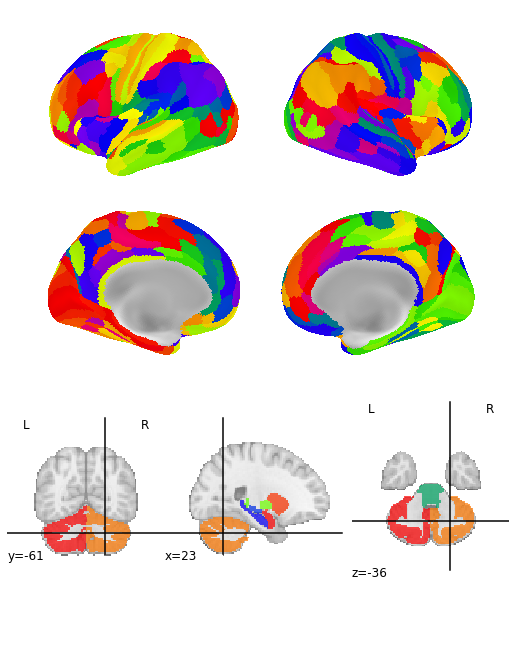